练习:将图形类的获取周长和获取面积函数设置成虚函数,完成多态
再定义一个全局函数,能够在该函数中实现:无论传递任何图形,都可以输出传递的图形的周长和面积
#include <iostream>using namespace std;
class Shape
{
protected:double ZC;double MJ;
public:Shape(double a,double b):ZC(a),MJ(b){}~Shape(){}Shape(const Shape &other):ZC(other.ZC),MJ(other.MJ){}Shape &operator=(const Shape&other){if(this!=&other){this->ZC=other.ZC;this->MJ=other.MJ;}return *this;}virtual double get_zc(){return ZC;}virtual double get_mj(){return MJ;}void show(){cout<<"矩形周长"<<ZC<<endl;cout<<"矩形面积"<<MJ<<endl;}
};
class Jx:public Shape
{
private:double k;double g;
public:Jx(int c,int d):Shape(2*(c+d),c*d),k(c),g(d){cout<<"矩形构造"<<endl;}~Jx(){cout<<"矩形析构"<<endl;}Jx(const Jx& other):Shape(other),k(other.k),g(other.g){cout<<"矩形拷贝构造"<<endl;}Jx &operator=(const Jx&other){if(this!=&other){Shape::operator=(other);this->k=other.k;this->g=other.g;}cout<<"矩形拷贝赋值"<<endl;return *this;}void show(){cout<<"矩形::k="<<k<<" g="<<g<<endl;cout<<"矩形周长"<<ZC<<endl;cout<<"矩形面积"<<MJ<<endl;}double get_zc(){return ZC;}double get_mj(){return MJ;}
};
class Yuan:public Shape
{
private:int r;
public:Yuan(int c):Shape(3.14*c,3.14*c*c),r(c){cout<<"圆构造"<<endl;}~Yuan(){cout<<"圆析构"<<endl;}Yuan(const Yuan& other):Shape(other),r(other.r){cout<<"圆形拷贝构造"<<endl;}Yuan &operator=(const Yuan&other){if(this!=&other){Shape::operator=(other);this->r=other.r;}cout<<"圆形拷贝赋值"<<endl;return *this;}void show(){cout<<"圆形::r="<<r<<endl;}double get_zc(){return ZC;}double get_mj(){return MJ;}};
void display(Shape &s)
{cout<<"面积:"<<s.get_mj()<<" 周长"<<s.get_zc()<<endl;
}
int main()
{Jx s1(2,8); //构造s1.show(); //调用的是继承下来的show函数cout<<"********************************"<<endl;Shape *ptr=&s1;ptr->show();cout<<"********************************"<<endl;display(s1);cout<<"********************************"<<endl;Yuan r1(2); //构造r1.show(); //调用的是继承下来的show函数cout<<"********************************"<<endl;Shape *ptr2=&r1;ptr2->show();cout<<"********************************"<<endl;display(r1);cout<<"********************************"<<endl;return 0;
}
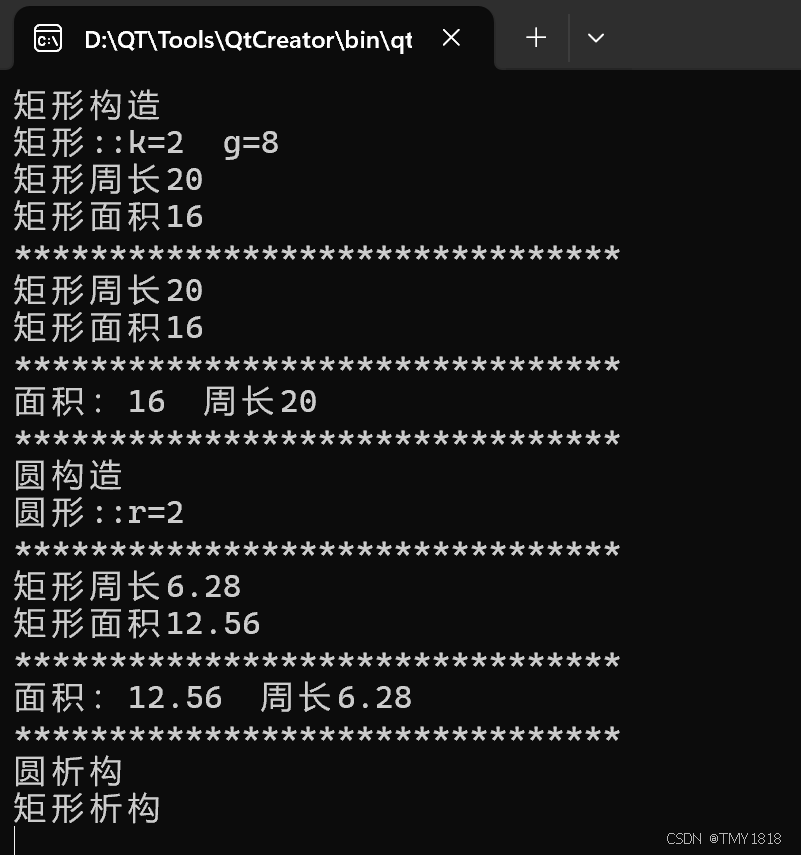
2> 手动实现一个循环顺序队列
#include <iostream>
using namespace std;class SeqQueue
{
private:int *table; //顺序队列指针int head; //队头下标int tail; //队尾下标int lenth; //实际长度int size; //队容量
public://无参构造SeqQueue():table(new int[100]), head(0), tail(0), lenth(0), size(100) {}//有参构造SeqQueue(int size):table(new int[size]) ,head(0), tail(0), lenth(0), size(size){}//析构函数~SeqQueue(){delete [] table;}//拷贝构造函数SeqQueue(const SeqQueue &R):table(new int[R.size]), head(R.head), tail(R.tail), lenth(R.lenth), size(R.size){//拷贝数据for(int i = 0; i < lenth; i++){this->table[(head+i)%size] = R.table[(head+i)%size];}}//拷贝赋值函数SeqQueue &operator=(const SeqQueue &R){//申请与拷贝源相同大小的内存空间delete [] this->table;this->table = new int[R.size];//拷贝数据this->head = R.head;this->size = R.size;this->tail = R.tail;this->lenth = R.lenth;for(int i = 0; i < lenth; i++){this->table[(head+i)%size] = R.table[(head+i)%size];}return *this;}//访问第一个元素int &front(){return this->table[this->head];}//访问最后一个元素int &back(){return this->table[this->tail-1];}//判空bool is_empty(){if(this->head == this->tail){return true;}else{return false;}}//判满bool is_full(){if((this->tail+1)%size == this->head){return true;}else{return false;}}//查看队列容量大小int get_size(){return this->size;}//查看队列实际长度int get_lenth(){return lenth;}//尾插一个元素void push(int e){if(is_full()){cout << "队列已满" << endl;return;}else{this->table[this->tail] = e;this->tail = (this->tail+1)%size;this->lenth++;}}//修改最后一个元素void emplace(int e){this->table[this->tail-1] = e;}//删除首个元素void pop(){if(is_empty()){cout << "队列已空" << endl;return;}else{this->head = (this->head+1)%size;this->lenth--;}}//展示void show(){int count = 0;for(int i = 0; i < lenth; i++){cout << this->table[(head+i)%size] << " ";count++;if(count % 5 == 0){cout << endl;}}if(count % 5 != 0){cout << endl;}}
};int main()
{SeqQueue A;for(int i = 0; i < 10; i++){A.push(i);}cout << "初始队列:" << endl;A.show();cout << "出队3个元素:" << endl;for(int i = 0; i < 3; i++){A.pop();}A.show();SeqQueue B = A;cout << "将A的数据拷贝给B" << endl;B.show();cout << "修改B的最后一个元素" << endl;B.emplace(100);B.show();cout << "其中" << endl;cout << "第一个元素:" << B.front() << endl;cout << "最后一个元素:" << B.back() << endl;cout << "队列容量:" << B.get_size() << endl;cout << "队列长度:" << B.get_lenth() << endl;return 0;
}
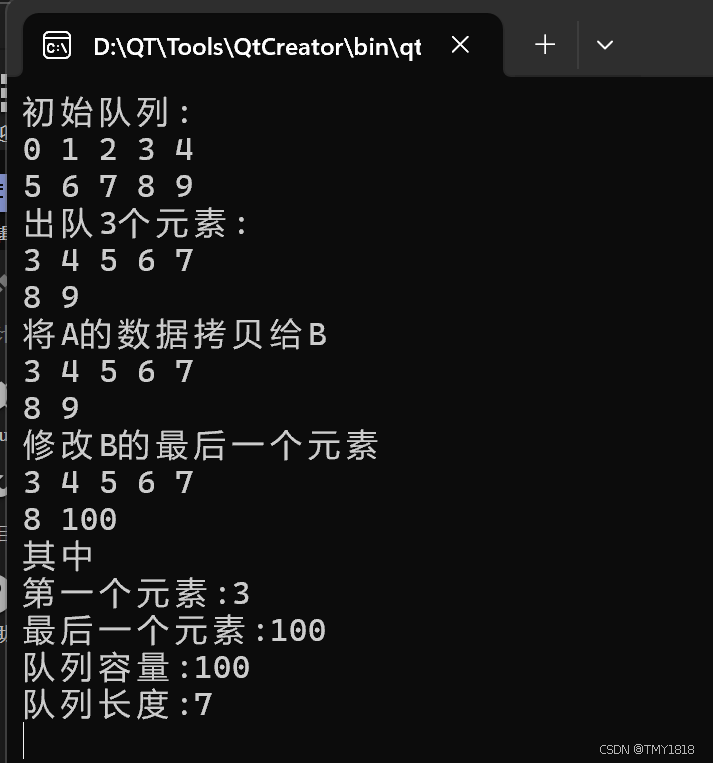
X-Mind
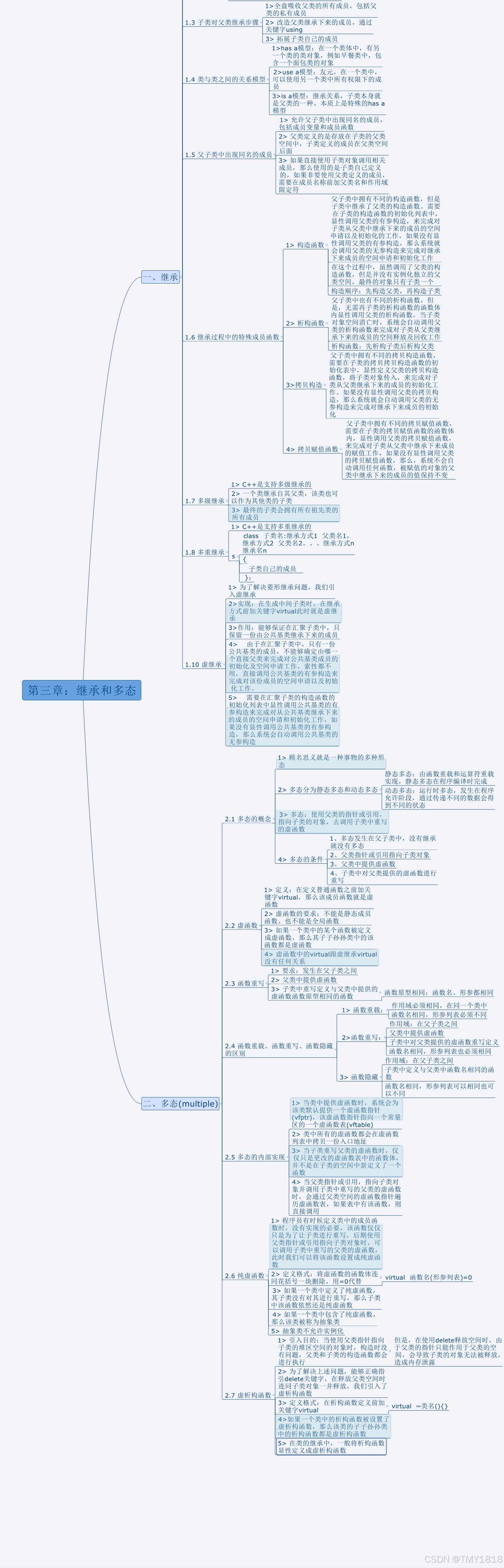